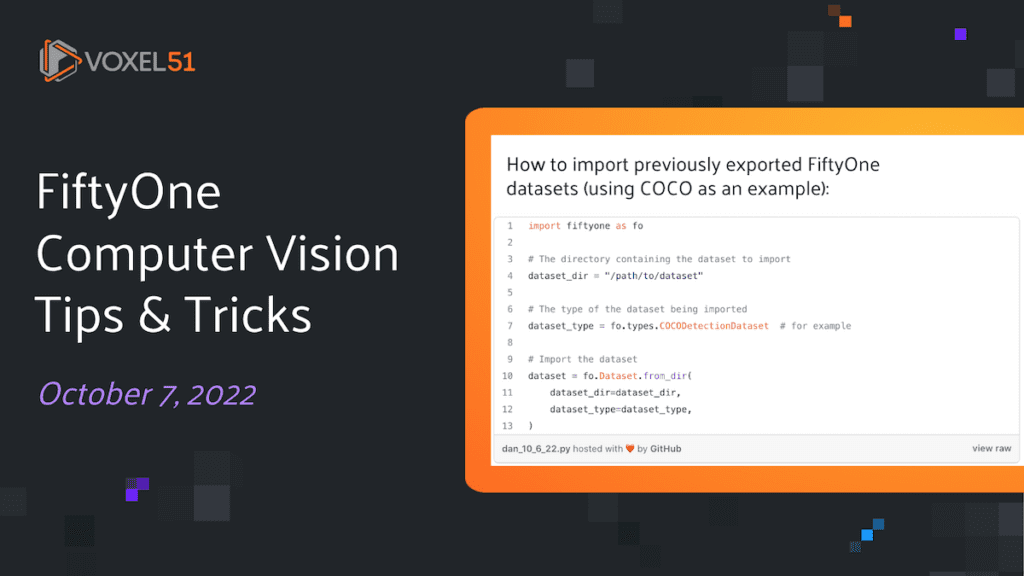
Welcome to our weekly FiftyOne tips and tricks blog where we recap interesting questions and answers that have recently popped up on Slack, GitHub, Stack Overflow, and Reddit.
Wait, what’s FiftyOne?
FiftyOne is an open source machine learning toolset that enables data science teams to improve the performance of their computer vision models by helping them curate high quality datasets, evaluate models, find mistakes, visualize embeddings, and get to production faster.
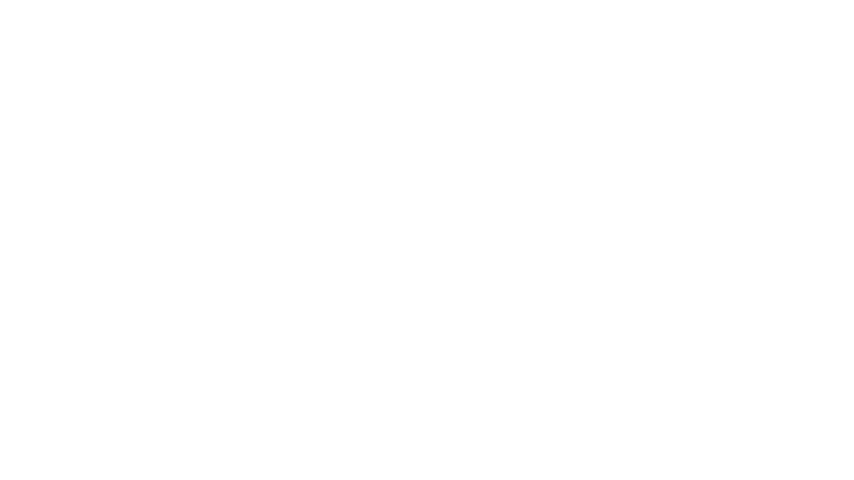
- If you like what you see on GitHub, give the project a star
- Get started! We’ve made it easy to get up and running in a few minutes
- Join the FiftyOne Slack community, we’re always happy to help
Ok, let’s dive into this week’s tips and tricks!
Importing previously exported FiftyOne datasets
Community Slack member Dan Erez asked,
“How can I import previously exported FiftyOne datasets?”
The basic syntax is:
import fiftyone as fo
# The directory containing the dataset to import
dataset_dir = "/path/to/dataset"
# The type of the dataset being imported
dataset_type = fo.types.COCODetectionDataset # for example
# Import the dataset
dataset = fo.Dataset.from_dir(
dataset_dir=dataset_dir,
dataset_type=dataset_type,
)
Learn more about loading datasets from disk in the FiftyOne Docs.
Support for TIFF images in the FiftyOne App
Stackoverflow member Joanna B asked,
“Can I load TIFF images using FiftyOne and Python in .ipynb notebook?”
The image types that FiftyOne supports are based on the underlying support of the browser you are using to run the FiftyOne App. The TIFF format is only supported out of the box by a few browsers like Safari. For other browsers, you will need to check if there is an extension that adds TIFF support, like this one for Firefox.
Learn more about the image types supported in FiftyOne in the FiftyOne Docs.
MSCOCO analysis on FiftyOne datasets
Community Slack member Sidney Guaro asked,
“Does Fiftyone have a MSCOCO analysis implementation that include things like localization error, background error, and similarity?”
Yes! By default, evaluate_detections()
will use COCO-style evaluation to analyze predictions when the specified label fields are Detections
or Polylines
. Although FiftyOne doesn’t directly expose a function that computes these metrics, you can construct dataset views that will allow you to compute many of these cases. For example stratifying by bounding box area. Another option is to export the relevant labels in COCO format and pass them to pycocotools.
Learn more about COCO-style evaluation in the FiftyOne Docs.
Working with absolute and relative paths
Community Slack member Teemu Sormuen asked,
“I can’t seem to reference images correctly due to issues with the root path. Currently, it seems that Sample.filepath
should be an absolute path, instead of a relative path with regards to the current working directory. I would like to use the current working directory as a relative base path for Sample.filepath
. Is there some way to define the relative path to which Sample.filepath
is compared to?”
The best way to do that is to modify the filepaths based on your current working directory. However, you can also use dataset.set_field()
to create a temporary view that updates filepaths using a ViewExpression
rather than changing them permanently with set_values()
each time. For example:
from fiftyone import ViewField as F
from fiftyone import ViewExpression as E
view = dataset.set_field(
"filepath",
E("/path/to/dir/").concat(F("filepath").split("/")[-1]),
)
Learn more about ViewExpressions in the FiftyOne Docs.
Importing and exporting custom datasets
Community Slack member Sagar Kalburgi asked,
“Can FiftyOne import a dataset that has been labeled using the LabelMe tool? Furthermore, is it possible to export the dataset in a different format after importing?”
Yes! You can always import custom labeled data into FiftyOne through a simple Python loop (here’s how to load custom label types like detections and instance segmentations), or by writing a custom dataset importer.
Once your data is loaded, you can then export it over two dozen formats.
Learn more about exporting datasets in different formats in the FiftyOne Docs.
What’s next?
- If you like what you see on GitHub, give the project a star
- Get started! We’ve made it easy to get up and running in a few minutes
- Join the FiftyOne Slack community, we’re always happy to help